We’ll be using the GeoIP2 PHP library from Maxmind. They have 2 versions: free and paid. We’ll be using the free version here.
- GeoIP Pros:
- The databases are located locally (on your server). So everything works fast.
- GeoIP Cons:
- The free version is under the CC license. So it requires attribution.
Please notice: The other solution (to get your visitors country from their IP address), would be to use an external service. For example, geoplugin.com. Please see this post for a detailed solution.
But, in my experience, when an external service can not be accessed, and you are sending a server-side request from PHP, your site load time could increase significantly. I saw when the page load time increased to ~2 minutes.
An external service could be down or working slowly. Or maybe you have a network problem between your server and theirs.
With the GeoIP solution described below, you wouldn’t have such problems.
Getting Country From IP With GeoIP2
1. Create a free account.
2. Download the prefilled configuration file GeoIP.conf
:
1) Log in to your account on maxmind.com. Click the item Automatic Updates in the left navigation:
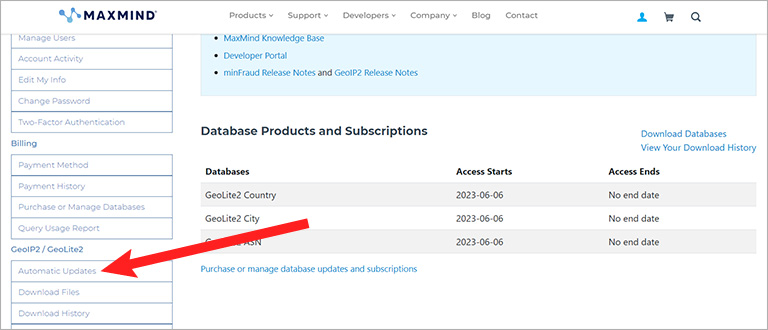
2) On the page that opens, download the pre-filled configuration file:
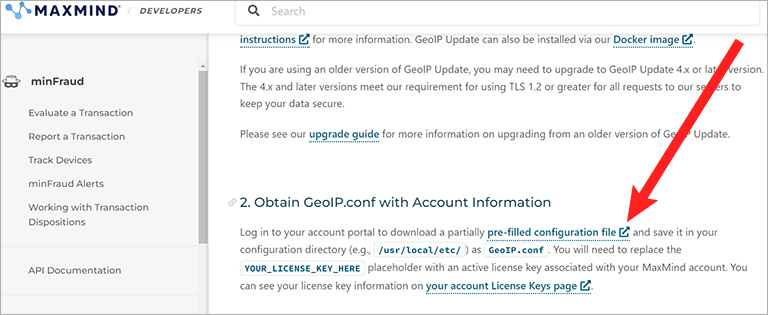
3. Install the GeoIP Update program:
sudo add-apt-repository ppa:maxmind/ppa sudo apt update sudo apt install geoipupdate
(source)
Please notice: if the command
sudo add-apt-repository ppa:maxmind/ppa
shows the error:
sudo: add-apt-repository: command not found
, it is necessary to install the software-properties-common
Ubuntu package:
sudo apt install software-properties-common
(source)
4. After installation, the GeoIP configuration file will be located in: /etc/GeoIP.conf
In this file, replace (from GeoIP.conf
downloaded by you on step #2) the following values:
- AccountID
- LicenseKey
- EditionIDs
5. Run from the terminal:
geoipupdate
After this, the directory /usr/share/GeoIP/
will contain 3 databases:
- GeoLite2-Country.mmdb
- GeoLite2-City.mmdb
- GeoLite2-ASN.mmdb
Please notice: to avoid running the command
geoipupdate
manually to update the databases, you can add a cronjob. For example, if you add this line to your crontab
file:
30 0 * * * /usr/bin/geoipupdate
, the update will run daily at 0:30 am.
6. Add the GeoIP2 library to your PHP project via Composer:
composer require geoip2/geoip2:~2.0
If you do not know how to install Composer, you can find the information here.
7. Get the country code and country name:
<?php require_once 'vendor/autoload.php'; use GeoIp2\Database\Reader; try { $reader = new Reader('/usr/share/GeoIP/GeoLite2-City.mmdb'); $record = $reader->city('165.227.97.17'); echo $record->country->isoCode . "\n"; // will output 'US' echo $record->country->name; // will output 'United States' } catch (\Exception $e) { ; // Handle exceptions here }
(source)
More examples could be found in the official documentation.